2022年05月13日
Agora.io VideoSDKの画面共有(ScreenSharing)実装例(その2)
※2020年の記事です。
現在、Agora.io VideoSDK 画面共有(ScreenSharing)については、サンプルが用意されています。
上記サンプルには、画面共有(ScreenSharing)と映像を両方表示するように実装されていないため、今回は、両方を表示できるサンプルに改造します。
Githubに公開しています。
目次
[非表示]機能一覧
※追加・変更機能のみ表示
[追加]
・カメラデバイス選択プルダウン →映像用
・マイクデバイス選択プルダウン →マイク用
・CAMERA_JOINボタン →映像のjoinと表示
・CAMERA_LEAVEボタン →映像のleave
・SCREENSHARINGボタン →(join後の)画面共有ダイアログ起動
開発環境
・S VideoSDK(Web)
Agora.io VideoSDK 映像と画面共有の両方を表示する実装例
実装のポイント
映像、画面共有(ScreenSharing)の両方を表示するには、映像、画面共有がそれぞれjoinし、streamを作成することがポイントです。
各種デバイス選択の追加
はじめに、カメラデバイスの選択、マイクデバイスの選択プルダウンを追加します。
<div>
<div>
<select name="microphoneId" id="microphoneId"></select>
</div>
<div>
<select name="cameraId" id="cameraId"></select>
</div>
</div>
各種ボタンの追加
次に、今回追加するボタンを追加します。
<button class="btn btn-raised btn-primary waves-effect waves-light" id="camera_join">CAMERA_JOIN</button>
<button class="btn btn-raised btn-primary waves-effect waves-light" id="camera_leave">CAMERA_LEAVE</button>
<button class="btn btn-raised btn-primary waves-effect waves-light" id="screensharing">SCREENSHARING</button>
各種ボタンクリック時
次に、今回追加するボタンをクリックした時の処理を追加します。
$("#camera_join").on("click", function (e) {
e.preventDefault();
console.log("camera_join")
const params = serializeFormData();
if (validator(params, fields)) {
rtc.camera_join(params);
}
})
$("#camera_leave").on("click", function (e) {
e.preventDefault();
console.log("camera_leave")
const params = serializeFormData();
if (validator(params, fields)) {
rtc.camera_leave();
}
})
$("#screensharing").on("click", function (e) {
e.preventDefault();
console.log("screensharing")
const params = serializeFormData();
if (validator(params, fields)) {
rtc.screensharing(params);
}
})
CAMERA_JOINボタンクリック時の詳細処理
camera_join(data){
console.log("camera_join");
this._client2 = AgoraRTC.createClient({mode: data.mode, codec: data.codec});
this._params2 = data;
// init client
this._client2.init(data.appID, () => {
console.log("init success");
this._client2.join(data.token ? data.token : null, data.channel, data.uid ? data.uid : null, (uid) => {
this._params2.uid = uid;
this._uid2 = uid;
Toast.notice("join channel: " + data.channel + " success, uid: " + uid);
console.log("join channel: " + data.channel + " success, uid: " + uid);
this._joined2 = true;
console.log(data.microphoneId);
console.log(data.cameraId);
this._localStream2 = AgoraRTC.createStream({streamID: uid, audio: true, cameraId: data.cameraId, microphoneId: data.microphoneId, video: true, screen: false});
this._localStream2.setVideoProfile('720p_3');
// The user has granted access to the camera and mic.
this._localStream2.on("accessAllowed",() => {
console.log("accessAllowed");
});
// The user has denied access to the camera and mic.
this._localStream2.on("accessDenied", () => {
console.log("accessDenied");
});
this._localStream2.init (() => {
console.log("getUserMedia successfully");
this._localStream2.play("local_stream", {fit: "cover"})
this._client2.publish(this._localStream2, (err) => {
console.log("Publish local stream error: " + err);
});
this._client2.on('stream-published', (evt) => {
console.log("Publish local stream successfully");
});
}, (err) => {
console.log("getUserMedia failed", err);
})
}, (err) => {
Toast.error("client join failed, please open console see more detail")
console.error("client join failed", err)
})
}, (err) => {
Toast.error("client init failed, please open console see more detail")
console.error(err);
});
}
CAMERA_LEAVEボタンクリック時の詳細処理
camera_leave () {
this._client2.leave(() => {
// close stream
this._localStream2.close();
// stop stream
this._localStream2.stop();
while (this._remoteStreams.length > 0) {
const stream = this._remoteStreams.shift();
const id = stream.getId()
stream.stop();
removeView(id);
}
this._localStream2 = null;
this._remoteStreams = [];
this._client2 = null;
console.log("client2 leaves channel success");
Toast.notice("leave success")
}, (err) => {
console.log("channel leave failed");
Toast.error("leave success")
console.error(err);
})
}
SCREENSHARINGボタンクリック時の詳細処理
screensharing(data){
console.log("screensharing start");
// create local stream
const streamSpec = {
streamID: this._params.uid,
audio: false,
video: false,
screen: true,
microphoneId: data.microphoneId,
cameraId: data.cameraId
}
// Your firefox need use support mediaSource at least
if (isFirefox()) {
streamSpec.mediaSource = 'window';
} else if (!isCompatibleChrome()) {
// before chrome 72 need install chrome extensions
// You can download screen share plugin here https://chrome.google.com/webstore/detail/agora-web-screensharing/minllpmhdgpndnkomcoccfekfegnlikg
streamSpec.extensionId = 'minllpmhdgpndnkomcoccfekfegnlikg';
}
this._localStream = AgoraRTC.createStream(streamSpec);
// set screen sharing video resolution
if (data.screenShareResolution != 'default') {
this._localStream.setScreenProfile(data.screenShareResolution);
console.log("set screen profile", data.screenShareResolution);
}
// Occurs when sdk emit error
this._localStream.on("error", (evt) => {
Toast.error("error", JSON.stringify([evt]))
})
// Occurs when a you stop screen sharing
this._localStream.on("stopScreenSharing", (evt) => {
this._localStream.stop();
this._showProfile = false;
Toast.notice("stop screen sharing")
})
// init local stream
this._localStream.init(() => {
console.log("init local stream success");
// play stream with html element id "local_stream"
this._localStream.play("local_stream", {fit: "cover"})
// run callback
resolve();
}, (err) => {
Toast.error("stream init failed, please open console see more detail")
console.error("init local stream failed ", err);
if (isFirefox()) {
console.error('Failed to start screen sharing, maybe firefox not support mediaSource, please upgrade your firefox to latest version')
return;
}
if (!isCompatibleChrome()) {
console.error('Failed to start screen sharing, maybe chrome extension was not installed properly, you can get it from https://chrome.google.com/webstore/detail/minllpmhdgpndnkomcoccfekfegnlikg');
return;
}
})
console.log("screensharing success");
return;
}
※参考サイト
Agora.io VideoSDK 画面共有(ScreenSharing)実装例(その1)
動作確認
それでは動作確認してみます。
1.画面起動
画面を起動します。(npm install、npm run devを実行しlocalhost:8080を表示)
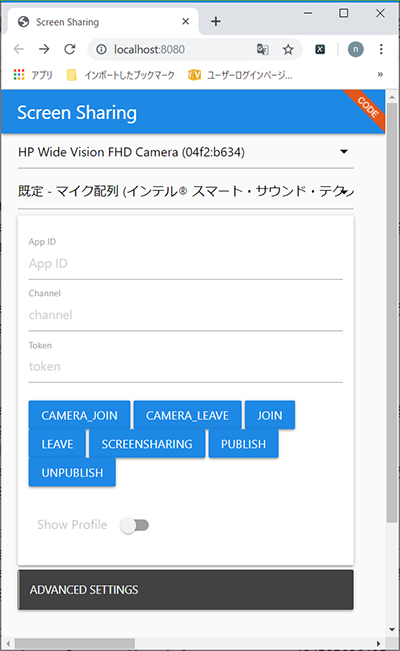
2.各種選択・入力
カメラ、マイクを選択し、appId、channelを入力します。
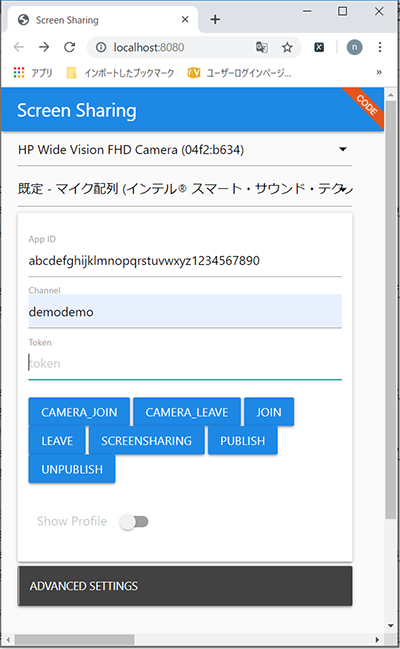
3.カメラ映像のJoin
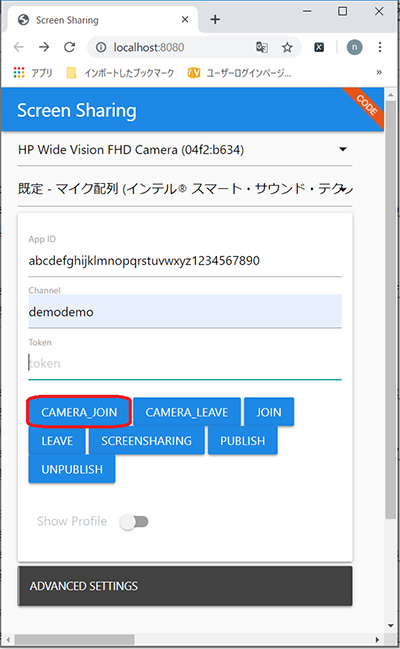
映像が表示されます。
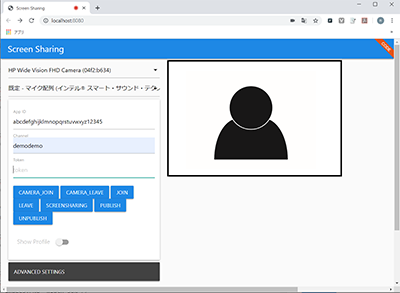
(注)イメージの映像は実際のカメラ映像ではありません。
4.画面共有分のJoin
Joinをクリックします。
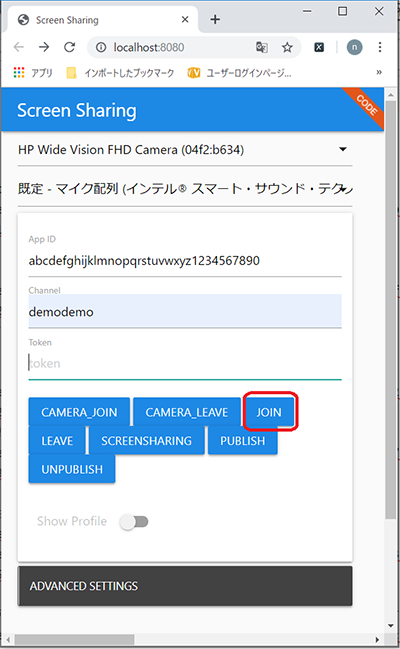
画面共有ダイアログが表示されます。(キャンセルして閉じておきます)
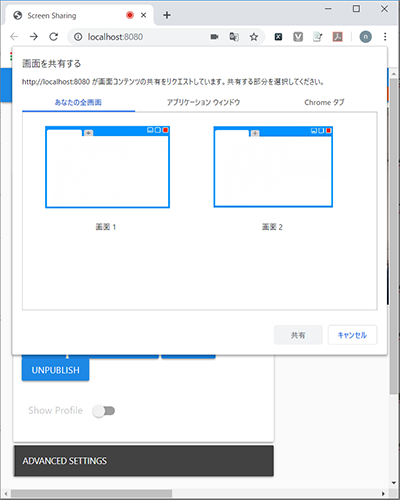
5.画面共有の再表示
SCREENSHARINGボタンをクリックし、再び画面共有ダイアログを起動します。
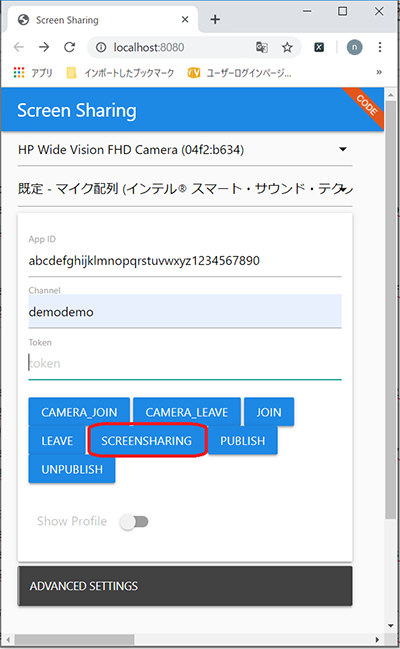
画面共有ダイアログが表示されます。
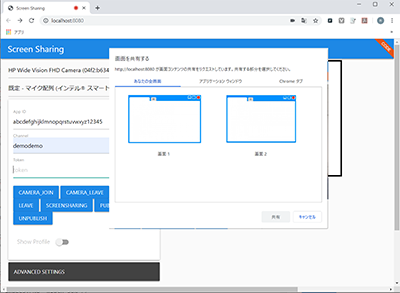
6.画面共有の開始
共有ボタンをクリックし、共有を開始します。
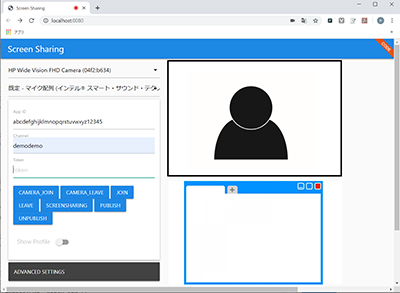
映像と画面共有を表示させることができました。
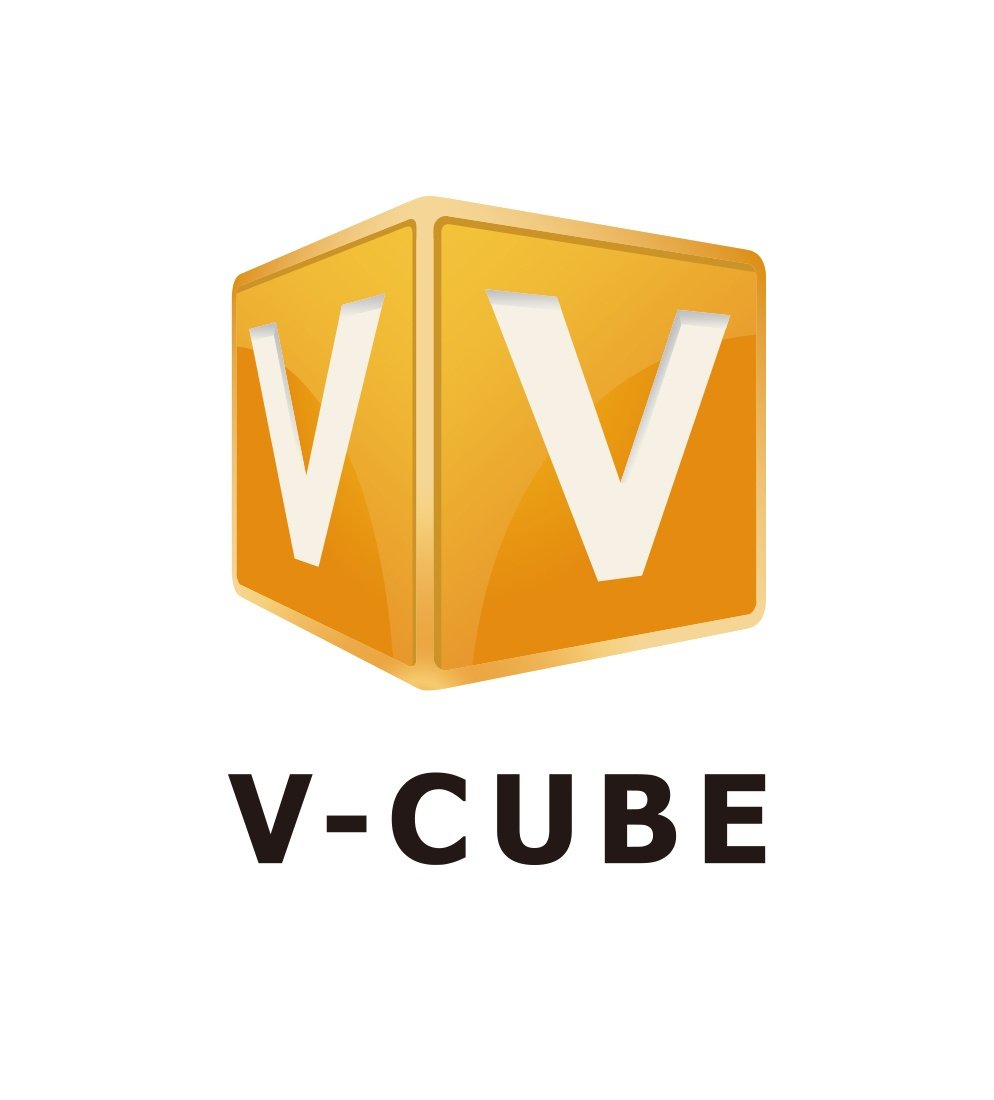
執筆者ブイキューブ